InputPopupUI
Overview
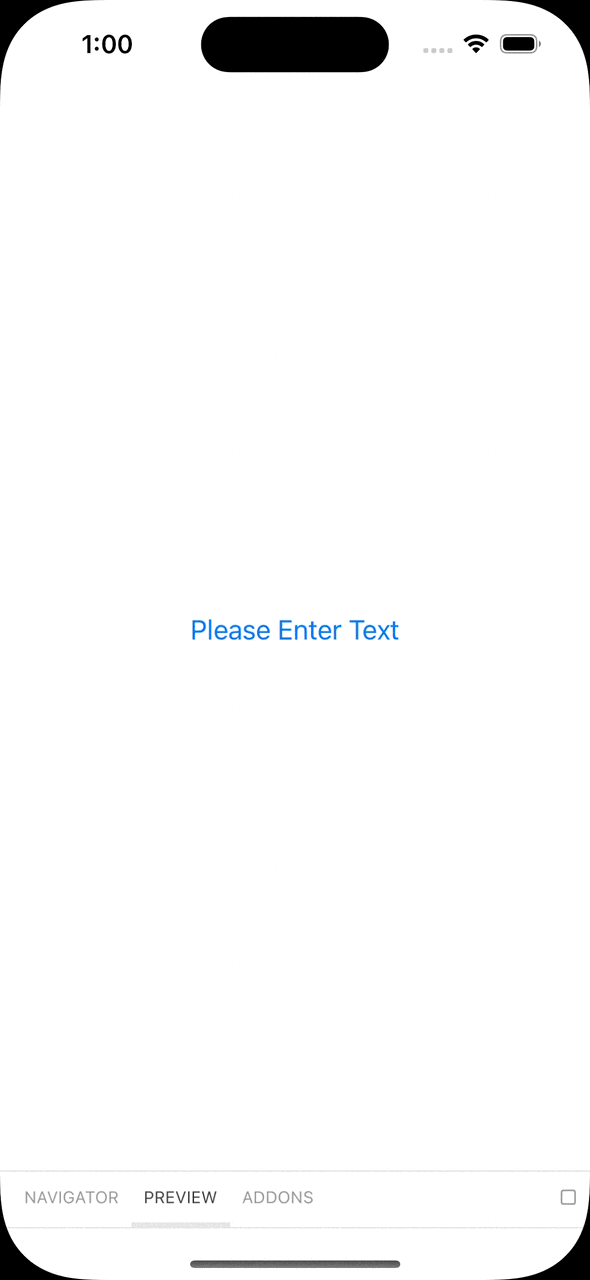
API Usage
Step 1. create popup
Using Default
InputPopup.tsx
import { InputPopupUI, createPopup } from 'react-native-global-components';
export default createPopup(InputPopupUI);
or you can override default props
InputPopup.tsx
import {
InputPopupUI,
InputPopupProps,
createPopup,
} from 'react-native-global-components';
const styles: InputPopupProps['styles'] = StyleSheet.create({
// override default styles
});
export default createPopup((props: InputPopupProps) => (
<InputPopupUI {...props} styles={styles} />
));
Step 2. register portal
App.tsx
const App = () => {
return (
<NavigationContainer>
<RootNavigator />
<InputPopup.Portal />
</NavigationContainer>
);
};
Step 3. use anywhere
InputPopup.show({
title: 'Hello👋🏻',
description: 'Please enter your name 🥰',
inputProps: { value: 'initial value' },
buttons: [
{ title: 'Cancel' },
{
title: 'Enter',
onPress: (text) => text && setText(text),
},
],
});
Props
title
title to show
Type | Default | Required |
---|---|---|
string | undefined | NO |
description
description text to show below title
Type | Default | Required |
---|---|---|
string | undefined | NO |
inputProps
react native text input props
Type | Default | Required |
---|---|---|
TextInputProps | undefined | NO |
buttons
react native button props with overriding onPress
. use renderButtons
for custom button UI.
Type | Default | Required |
---|---|---|
InputPopupButtonProps | [{ title: 'Cancel', color: 'black' },{ title: 'Confirm' },] | NO |
// ButtonProps from react native
interface InputPopupButtonProps extends Omit<ButtonProps, 'onPress'> {
onPress?: (text?: string) => void;
}
renderButtons
render function for Headless button UI. buttons
will be ignored when renderButtons
provided.
Type | Default | Required |
---|---|---|
(text?: string) => ReactElement | undefined | NO |
fadeAnimationConfig
fade animation configuration. see useFadeAnimationStyle.
Type | Default | Required |
---|---|---|
FadeAnimationConfigs | undefined | NO |
androidBackBehavior
behavior on android hardware back button pressed. hide component for default.
Type | Default | Required |
---|---|---|
'hide' or 'none' | 'hide' | NO |
KeyboardAvoidingLayoutProps
keyboard related props overriding react native KeyboardAvoidingViewProps
Type | Default | Required |
---|---|---|
KeyboardAvoidingLayoutProps | undefined | NO |
interface KeyboardAvoidingLayoutProps extends KeyboardAvoidingViewProps {
bottomInset?: number;
topInset?: number;
children?: React.ReactNode;
}
styles
style object to override default style
Type | Default | Required |
---|---|---|
Styles | undefined | NO |
type Styles = {
title?: StyleProp<TextStyle>;
input?: StyleProp<TextStyle>;
description?: StyleProp<TextStyle>;
container?: StyleProp<ViewStyle>;
buttonContainer?: StyleProp<ViewStyle>;
};