AlertPopupUI
Overview
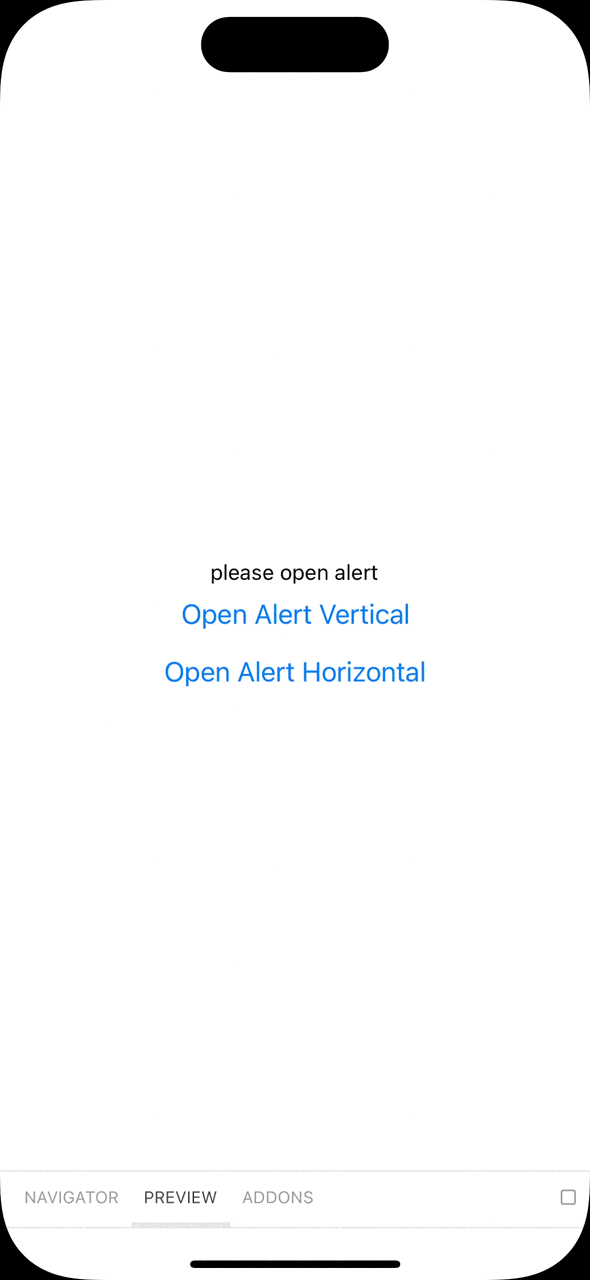
API Usage
Step 1. create popup
Using Default
AlertPopup.tsx
import { AlertPopupUI, createPopup } from 'react-native-global-components';
export default createPopup(AlertPopupUI);
or you can override default props
AlertPopup.tsx
import {
AlertPopupUI,
AlertPopupProps,
createPopup,
} from 'react-native-global-components';
const styles: AlertPopupProps['styles'] = StyleSheet.create({
// override default styles
});
export default createPopup((props: AlertPopupProps) => (
<AlertPopupUI {...props} styles={styles} />
));
Step 2. register portal
App.tsx
const App = () => {
return (
<NavigationContainer>
<RootNavigator />
<AlertPopup.Portal />
</NavigationContainer>
);
};
Step 3. use anywhere
AlertPopup.show({
title: `Hi I'm Vertical Popup`,
message: 'select how do you feel today',
vertical: true,
options: [
{
text: 'Good 😝',
},
{
text: 'Not Okay 😢',
color: 'green',
},
{
text: `Don't ask me 😡`,
color: 'red',
},
],
});
Props
message
message to show
Type | Default | Required |
---|---|---|
string | undefined | YES |
title
title text to show above message
Type | Default | Required |
---|---|---|
string | undefined | NO |
options
options buttons to show. see option interface below
Type | Default | Required |
---|---|---|
AlertPopupOption[] | [{ text: 'Ok' }] | NO |
interface AlertPopupOption {
text: string;
onPress?: (text: string) => void;
color?: string;
textStyle?: StyleProp<TextStyle>;
testID?: string;
}
touchableOpacityProps
touchableOpacity props of button to override default settings
Type | Default | Required |
---|---|---|
TouchableOpacityProps | undefined | NO |
vertical
flag to render options vertically or horizontally
Type | Default | Required |
---|---|---|
boolean | false | NO |
fadeAnimationConfig
fade animation configuration. see useFadeAnimationStyle.
Type | Default | Required |
---|---|---|
FadeAnimationConfigs | undefined | NO |
headerElement
header content to render above title
Type | Default | Required |
---|---|---|
ReactElement | undefined | NO |
footerElement
footer content to render below message
Type | Default | Required |
---|---|---|
ReactElement | undefined | NO |
styles
style object to override default style
Type | Default | Required |
---|---|---|
Styles | undefined | NO |
interface Styles {
option?: StyleProp<ViewStyle>;
container?: StyleProp<ViewStyle>;
message?: StyleProp<TextStyle>;
title?: StyleProp<TextStyle>;
optionContainer?: StyleProp<ViewStyle>;
messageContainer?: StyleProp<ViewStyle>;
}