createPopup ⭐️
Example
// AlertPopup.tsx
createPopup(AlertPopupUI, { shouldWaitForUserInteraction: true });
// other file
await AlertPopup.show(props);
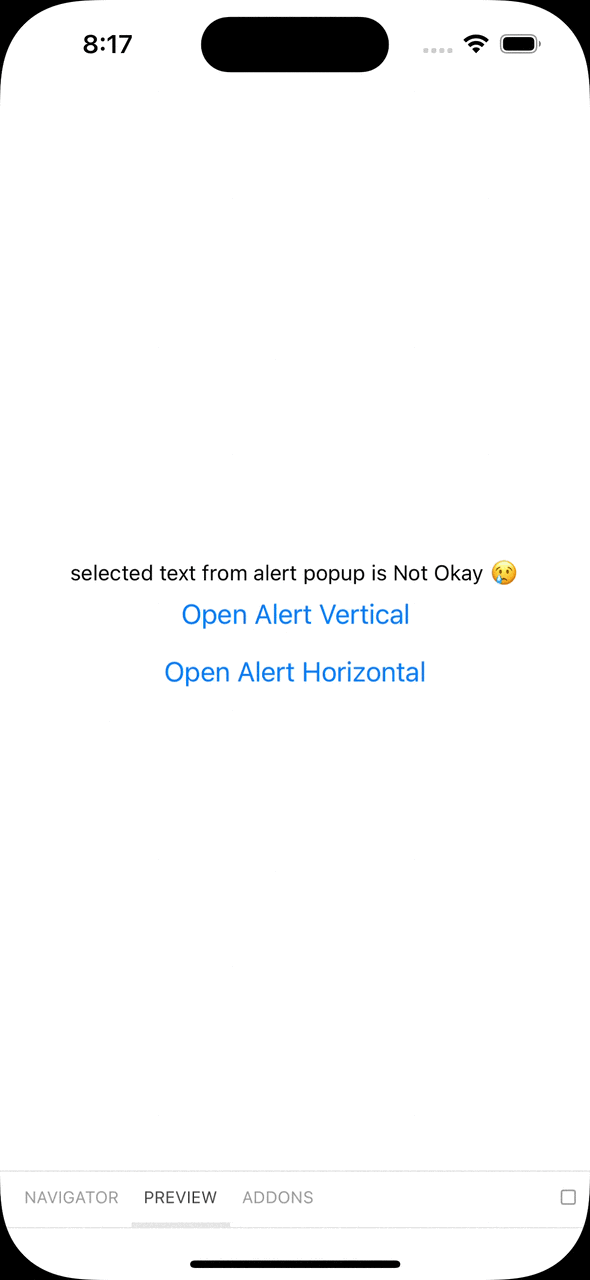
PopupOptions
createPopup(SimpleSnackbarUI, { shouldWaitForUserInteraction: true });
shouldWaitForUserInteraction
if shouldWaitForUserInteraction
set to true,
multiple popup show request will wait for currently active popup to hide then show
if set to false, currently active popup will hide immediately
Type | Default | Required |
---|---|---|
boolean | false | NO |
Usage
Step 1. create popup
First, implement your custom UI or you can use any PopupUIs we provide
You might need core hooks for state management. see usePopupContext
MyPopup.tsx
import { createPopup } from 'react-native-global-components';
interface MyPopupProps {
name: string;
}
const MyPopup: FC<MyPopupProps> = (props) => {
return <View />;
};
export default createPopup(MyPopup);
Step 2. register portal
Portal is where your component actually rendered when you call show
method.
If using React Navigation, highly recommend to put it in NavigationContainer
to use navigation context in global components.
App.tsx
const App = () => {
return (
<NavigationContainer>
<RootNavigator />
<MyPopup.Portal />
<MySnackbar.Portal />
</NavigationContainer>
);
};
then you can do
MyPopup.tsx
import { useNavigation } from '@react-navigation/native';
import { createPopup, usePopupContext } from 'react-native-global-components';
const MyPopup: FC<MyPopupProps> = ({ name }) => {
const navigation = useNavigation();
const { hide } = usePopupContext();
return (
<Button
onPress={() => {
navigation.navigate('MyProfileScreen', { name });
hide();
}}
/>
);
};
export default createPopup(MyPopup);
Step 3. use anywhere
await MyPopup.show({
name: 'Jerry and Zeki',
});
Methods
show
method to show created global component
generic T
(MyPopupProps
for this guide) is inferred from your component props
type show = (props: T) => Promise<void>;
hide
method to hide currently visible global component
type hide = () => Promise<void>;
Portal
function component to render global component
type Portal = React.FC<{}>;